The javascript ecosystem has grown enormously over the last decade. It has evolved from developing client applications to building desktop and mobile applications. Javascript has become inevitable regardless of the platform. One of the reasons being Node.js.
People often confuse Node.js with frameworks/programming languages. Node.js is a runtime environment that helps run javascript on servers. It takes the chrome v8 engine and compiles the javascript code on the servers.
Node.js helps developers build applications on server-side, desktop, and mobile applications. Having Node.js as the base, a lot of frameworks have evolved in recent years.
Here are some of the exciting facts about Node.js
- Some of the popular websites like Netflix,eBay, PayPal, and LinkedIn use Node.js in their application development
- 85% of developers use Node.js to develop web applications.
- Since Node.js uses javascript to build the backend, it’s easy for javascript developers to create a complete product.
Let’s look at some of the popular Node.js frameworks:
Types of Node.js Frameworks
There are different ways and patterns to develop a web application. In the modern era, we can create an application using Monolith architecture or build it as Microservices. Based on the architecture that we choose, it changes an application lifecycle. We can categorize the Node.js frameworks into three different styles.
REST API Frameworks
REST API is a standard that helps to transfer the resources using the HTTP protocol. There are different methods used in REST-based architecture. They are,
- GET - provides read-only access to the resources.
- POST - allows to add or store the resources.
- PUT - it provides an option to update the resources
- DELETE - it deletes or removes the resources.

A lot of frameworks in Node.js provide us the facility to develop REST APIs. Clients side like browsers or mobile, can consume the data and update the server-side using REST APIs.
MVC Frameworks
MVC Frameworks provides us scalable solution by splitting the layers as:
- Model
- View
- Controller
It makes the process easier by splitting the application layers as Model, View, and Controller and allows us to scale the application.
The way MVC Framework works is:
- Model updates the View
- Client/User uses View and uses a controller to update the data.
- The controller manipulates the Model to change the data in our database.
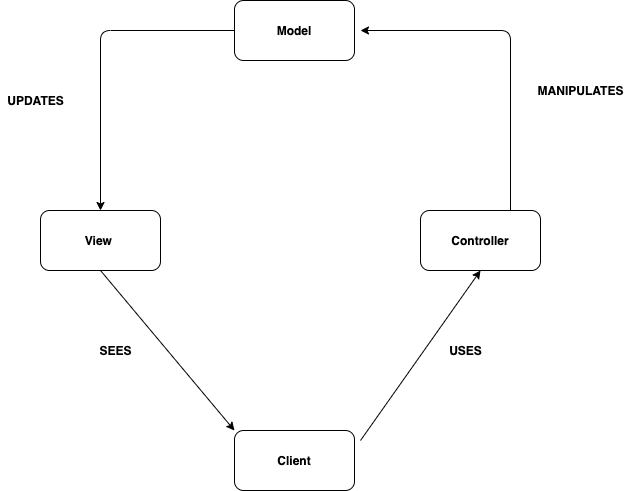
Full-Stack MVC Frameworks
Full Stack MVC Frameworks can take care of both the client-side and server-side of an application. It follows MVC Architecture with additional features such as scaffolding, template engine, middlewares, libraries and provides options to automate many repetitive tasks on application development.
It’s an MVC Framework with additional features on top of it. Choosing a full-stack MVC framework comes with a lot of advantages.
- It helps us to develop an application quickly.
- We can automate the repetitive tasks easily with Full stack MVC frameworks.
- Maintain a single codebase, and it can be managed efficiently by developers when the application grows.

Our List of the Most Prominent Node.js Frameworks: Features, Pros, and Cons
So far, we have seen the different categories of frameworks. According to Node.js survey reports, Node.js frameworks have increased productivity among developers by 68%, improves developer satisfaction, increased application performance, and reduced development costs. Let’s look into some of the most prominent node.js frameworks available in the industry.
ExpressJS
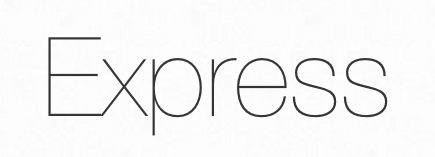
ExpressJS is one of the popular Node.js frameworks. It is a fast, minimalistic web framework within Node.js with features that help build robust API for mobile and web applications.
One of the main advantages of ExpressJS is the learning curve. It’s easy to learn and get started with it. In that way, beginners can quickly adapt and start working on applications using ExpressJS.
Features
- It provides middleware that can allow us to configure and write our business logic for HTTP requests.
- It provides high performance. Because of Node.js’ asynchronous nature, it helps us to handle multiple requests efficiently.
- It follows MVC architectural pattern that helps us to render HTML pages using template engines.
Pros
- Developing an application can be quick. We can build the MVP of a product in a brief period using ExpressJS.
- It handles requests efficiently with the help of I/O request handling techniques.
- Express is matured and has a highly supported open source community. It has a large number of packages for utilities.
- Easily integrates with third-party applications.
Cons
- ExpressJS has a drawback of callback hell.
- Express middleware can cause problems in client request handling.
- It doesn’t support much on the security aspects. It’s up to us to handle the application’s security.
Hapi.js
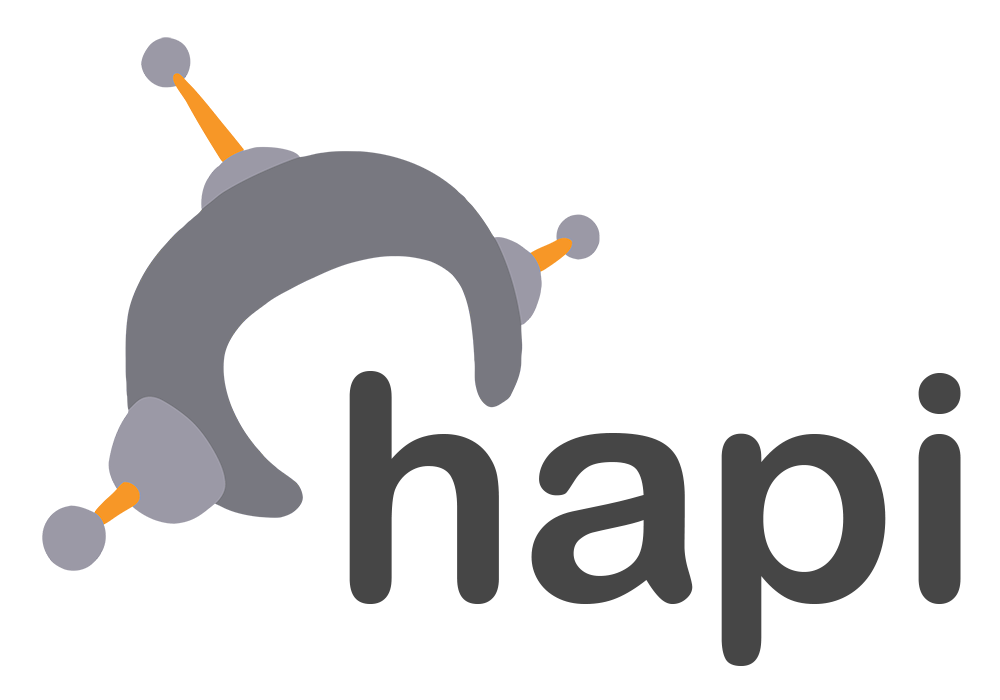
Hapi.js is a REST API-based framework to build scalable web applications and developed by the mobile development team of Walmart Labs. An interesting fact about Hapi.js is that it handled all the requests of Walmart’s mobile application on black Friday traffic with about 10 CPUs and 28 GB of RAM.
Features
- It provides reusable and cacheable functions that we can access throughout the application.
- It provides abstraction layers that help to break the application into several logical components.
- It follows zero routing conflict methodology. Paths will never conflict.
Pros
- It provides a robust and extendable plugin system that helps develop applications faster and efficiently.
- It comes with batteries included. It mainly provides modules like caching mechanism, authentication, and input validations out of the box.
- It has better control over request handling.
Cons
- There are no rules or official guidelines for structuring the codebase. So, It’s up to the developer to follow the standard. It can become a problem if it’s not strictly followed across codebases.
- Even though it comes with prebuilt modules, there is no way to Scaffold. Endpoints have to be created and tested manually.
- Since it’s tightly coupled with Hapi.js developing an application, it’s challenging to migrate to other frameworks.
Sails.js

Building an Enterprise-grade application from scratch can be difficult. We need to maintain code structure and design principles by ourselves across the company. A framework like Sails.js solves that problem for us. It is an MVC-based web application framework for enterprise-grade applications.
It resembles MVC architecture from a framework like ruby on rails. It follows the “convention over configuration” principle. It helps us to build Real-time applications quickly.
Features
- It uses ExpressJS under the hood.
- It provides WebSockets to build real-time applications out of the box. It’s well supported.
- It provides a waterline ORM/ODM, which makes it Database agnostic.
- Sails.js has powerful code generation. It has a concept called blueprint actions that helps to generate code efficiently.
- It prefers Conventions over configuration.
Pros
- MVC-based architecture. So, it separates business logic from UI.
- It provides free JSON API generation, and we don’t need extra routing.
- It allows us to store data anywhere. Since it uses waterline ORM/ODM, it’s database agnostic.
Cons
- Sails.js is slow with high memory consumption.
- Managing static assets on an application can become clumsy.
- Sometimes, building an application can be slow and become time-consuming. So, it takes time to boot up on the production server.
Koa.js
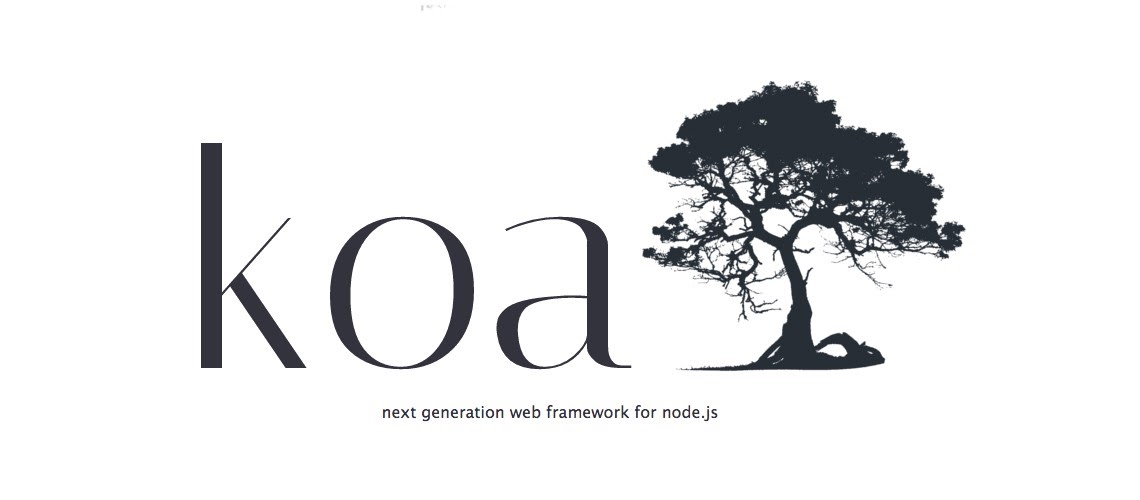
Koa.js is the latest web framework designed by the team behind Express. It raises the question of why there is a need for a new framework.
Well, koa is smaller and more expressive and packed with async functions by default. We’ve seen that Express has limitations with callbacks. So, the team decided to solve that problem with Koa.js by providing async tasks by default.
Features
- Koa is modern, future-proof, and built on ES6, a Modern javascript to build web applications easier.
- It uses an ES6 generator to create downstream or upstream functionalities. It helps us to avoid callback hell.
- It provides built-in catchall modules that handle the error effectively.
- It uses a context object, which is an encapsulation of request and response objects.
Pros
- Extremely light-weighted with just 550 lines of code.
- It uses a generator to avoid callbacks from the beginning.
Cons
- Using generators makes our application harder to migrate to other frameworks in the future. It creates a tight coupling with the framework.
- Koa has a small community when compared with ExpressJS.
- It’s not compatible with express style middlewares.
Meteor.js
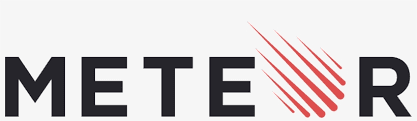
Meteor.js is a Full Stack javascript platform to build web and mobile applications. It offers a complete ecosystem to build applications so that we don’t need to combine different tools with building full-stack applications.
Features
- It provides a full-stack solution for developing web applications with several built-in features, templates, and hot reload.
- It has all the front-end and back-end components that help developers build the entire application quickly.
- It provides isomorphic javascript code. It avoids the need to configure different modules, libraries to perform the functionality.
- It provides hot reloading features for the application.
- Meteor has its custom package manager.
Pros
- No middleware or ORM is required to build backend solutions.
- Code reusability. We can use the same components to build mobile and web applications.
- It also provides support for graphql with a built-in MongoDB handler.
Cons
- Dependency on prebuilt packages can conflict once the application grows.
- It will be simpler to use Express if we want to build simple web applications. Sometimes, Meteor may feel bloated for particular use-cases.
Nest.js
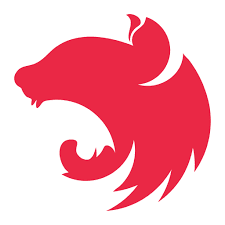
Nest.js is a progressive Node.js framework that helps to build efficient and scalable Node.js server-side applications. It’s using Express under the hood. One of the main advantages of Nest.js over Express is that it provides an exact architectural way that helps us build enterprise-grade applications quickly.
Features
- It supports typescript out of the box.
- It provides a powerful CLI to ease the development process.
- Nest.js provides us an option to build Microservices based applications out of the box.
- Well-maintained documentation.
Pros
- It can be scalable and follows a strict design principle like angular. It can benefit us when building enterprise-level applications.
- Code generation helps to develop applications faster.
- It uses the latest version of Typescript. So, it helps us in type checking.
Cons
- It’s more of Angular styled application development. So, it has chances that javascript developers may feel difficulty or lose interest over time.
- It has a steep learning curve.
- Community is small when compared with Express.
Socket.IO
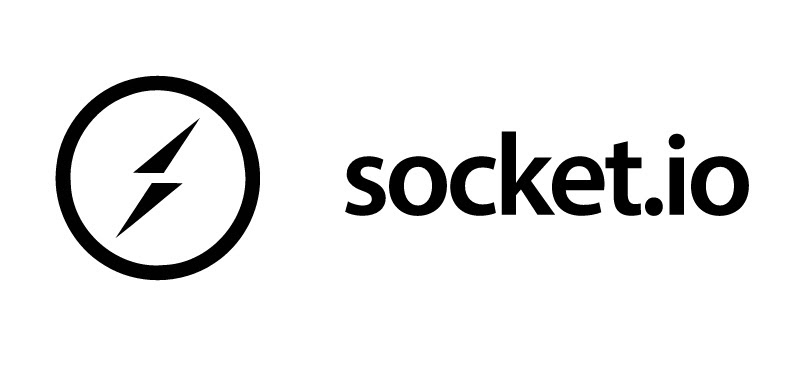
Even though SocketIO doesn’t come under a category of node js framework. It has become an inevitable library when it comes to building a real-time application using javascript.
It establishes a bi-directional communication between the client and server-side. One of the common use-cases of it is building chat applications like WhatsApp and Facebook messenger.
Features
- We can use SocketIO on both the client-side and server-side.
- It provides MultiPlexing support.
- SocketIO provides a reliable WebSocket connection for Node.js applications.
- It provides Error detection and auto-correction with auto reconnection support.
Pros
- It’s easy to get started with SocketIO. It helps to develop real-time applications quickly.
- It provides a more straightforward way to set up audio and video streaming functionalities for the application.
- It provides binary streaming for the application data.
Cons
- SocketIO is callback-centric which is a real downside. Callback doesn’t provide a guarantee on reliable data streaming.
- It also doesn’t provide a message guarantee to acknowledge that it received a message. We need to write custom logic in our application to handle such scenarios.
Adoni.js
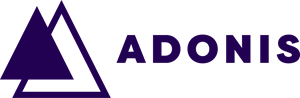
Adoni.js is an MVC-based framework that helps us build a stable webserver without worrying about packages and libraries. It helps us to focus only on the core business logic. It follows the same principles and concepts as Laravel.
Features
- We can choose to build Full Stack Applications or just REST API with Adoni.js.
- It has WebSocket support out of the box.
- It provides built-in modules for every functionality like Authentication, Mailing, routing, and social integrations.
Pros
- Easy to learn if you’re already familiar with the Laravel framework.
- It’s Opinionated in the right way for CRUD API.
- It has the “Write less code and achieve more” principle.
Cons
- Small community
- Documentation needs to be improved.
- Adoni.js lacks plugin support to cover various use-cases.
Loopback.js
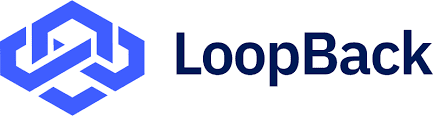
Loopback.js is a Node.js framework that helps us to build REST API with little or No coding. It uses ExpressJS under the hood. It can generate a fully functional end-to-end REST API for web applications.
Features
- Loopback gives you the power of Full Stack development. We can share the same model with both client and server-side.
- It provides built-in modules and features. We can create CRUDAPIs with simple commands.
- Scaffolding is an easy process, and it helps to develop applications faster.
Pros
- It provides a structured and organized codebase to build scalable applications.
- It provides swagger as API explorer. It comes in handy to test the APIs and share it across the team.
- It has built-in user and access role functionalities.
Cons
- It has a steep learning curve.
- Loopback has a small community. So, sometimes it will be hard to get support for issues or queries.
- It provides a monolithic architectural style. It can cause scalability issues when the application grows.
Total.js
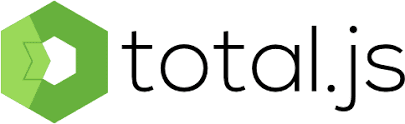
Total.js is a Node.js framework that offers a CMS-like experience to build web applications. It has a collection of libraries and UI components that help to develop a complete product.
Features
- It provides a client-side library called jComponents with pre-built components. It helps to build the front-end of the application.
- One of the important features of total.js is that it provides a code editor on the client environment. We can develop our application using the cloud-based editor.
- More than 230 UI components available in the framework.
Pros
- Low CPU and Memory consumption. It makes the application more efficient.
- It provides multiple database compatibility.
- Best known for real-time tracking in modern applications.
Cons
- It’s developed as a “One-man show” so, maintaining and building the whole application by small and medium applications.
- Documentation needs to be improved.
- Smaller community to get support for our application.
Derby.js

Derby.js is a full-stack MVC framework for writing a modern web application. It follows MVC architectural pattern and uses shareDB’s to provide real-time data synchronization between client and server.
It provides the facility to render HTML pages on the server-side. It uses a racer engine for real-time data synchronization.
Features
- It provides a Racer engine for real-time data synchronization across clients and servers.
- It has live binding between view and model in the application. So, it reflects any changes in the model instantly.
- It provides server rendering that helps to render HTML pages on the server-side.
Pros
- We can use the same code on both the client and server-side of the application.
- It provides faster communication between the client and server-side.
Cons
- Just like other frameworks, it’s has a smaller community. It can be a problem when it comes to supporting and queries.
- Poor documentation makes it hard to understand the concepts.
Feather.js

As the name suggests, it is a lightweight framework to build real-time applications and REST APIs using javascript or typescript.
Features
- It provides default plugins for standard modules like authentication and payment services.
- It provides reusable components across the client and server-side of our application.
- It provides CRUD API via services. Both REST API and real-time applications thought web sockets automatically.
Pros
- It’s lightweight
- Code reusability is a more significant advantage in feather.js. It saves a lot of development time and cost.
- CLI commands make the application scaffolding easier.
- It has good documentation that comes in handy while referring to the concepts and building applications.
- It has a lot of default plugins.
Cons
- Even though it’s not a significant disadvantage, it’s mainly to build REST API.
- It has minimal usage across the industry.
- Although documentation is good, it’s not easy to set up as a beginner.
Recap
With Node.js frameworks’ support, it has become effortless to build scalable applications in the modern world. It’s also essential to choose a framework based on our application requirements.
With Node.js frameworks’ support, it has become effortless to build scalable applications in the modern world. It’s also essential to choose a framework based on our application requirements or you can discuss with experts who provide application development services to get an honest opinion.